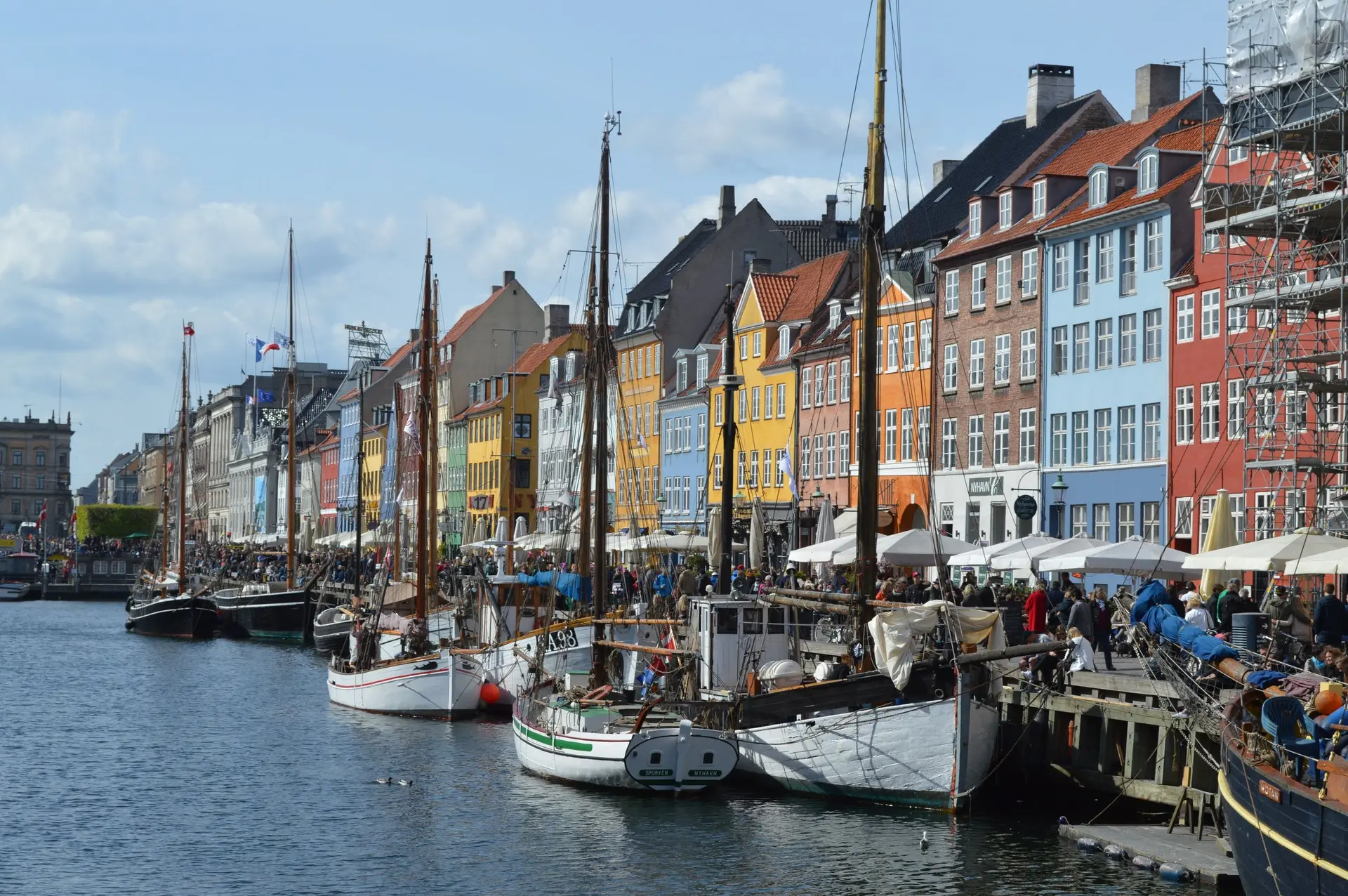
AWS API Gateway stages and ASP NET CORE Middleware
I am running three different Dynamo configurations in my ASP NET Core Razor Pages app. One for local developement where I am targeting localhost- running Dynamo in a docker container. One for API Gateway Stage Environment and one for Production Stage Environment.
In this post I will cover what I did to understand which API Gateway Stage the request is coming from so I could set a Table Prefix when using the Object Persistent Model approach with Dynamo and the AWS SDK
Read more about the High Level Dynamo SDK here.
I setup a middleware and inspect the LambdaContext where I can read the Stage Variables set up in API Gateway. If my stage variable "LambdaAlias" equals "Staging" I prefix my DynamoTable with "dev_". So it will now target my dev dynamo table in AWS.
See working code below. Remember to register the middleware in Startup.cs. You can use the extension method in the bottom of the code block.
using Amazon;
using Amazon.Lambda.APIGatewayEvents;
using Amazon.Lambda.AspNetCoreServer;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Logging;
using System;
using System.Threading.Tasks;
namespace MyApp.Middlewares
{
public class DynamoConfigurationMiddleware
{
private readonly RequestDelegate _next;
private readonly ILogger<DynamoConfigurationMiddleware> _logger;
public DynamoConfigurationMiddleware(RequestDelegate next, ILogger<DynamoConfigurationMiddleware> logger)
{
_logger = logger;
_next = next;
}
public Task Invoke(HttpContext httpContext)
{
try
{
var lambdaContext = (APIGatewayProxyRequest)httpContext.Items[APIGatewayProxyFunction.LAMBDA_REQUEST_OBJECT];
if (lambdaContext == null)
{
return _next(httpContext);
}
var stage = lambdaContext.StageVariables["LambdaAlias"];
if (stage?.Equals("Staging", StringComparison.InvariantCultureIgnoreCase) == true)
{
AWSConfigsDynamoDB.Context.TableNamePrefix = "dev_";
}
}
catch (Exception ex)
{
_logger.LogCritical("Middleware failed", ex);
}
return _next(httpContext);
}
}
public static class DynamoConfigurationMiddlewareExtensions
{
public static IApplicationBuilder UseDynamoConfigurationMiddleware(this IApplicationBuilder builder)
{
return builder.UseMiddleware<DynamoConfigurationMiddleware>();
}
}
}