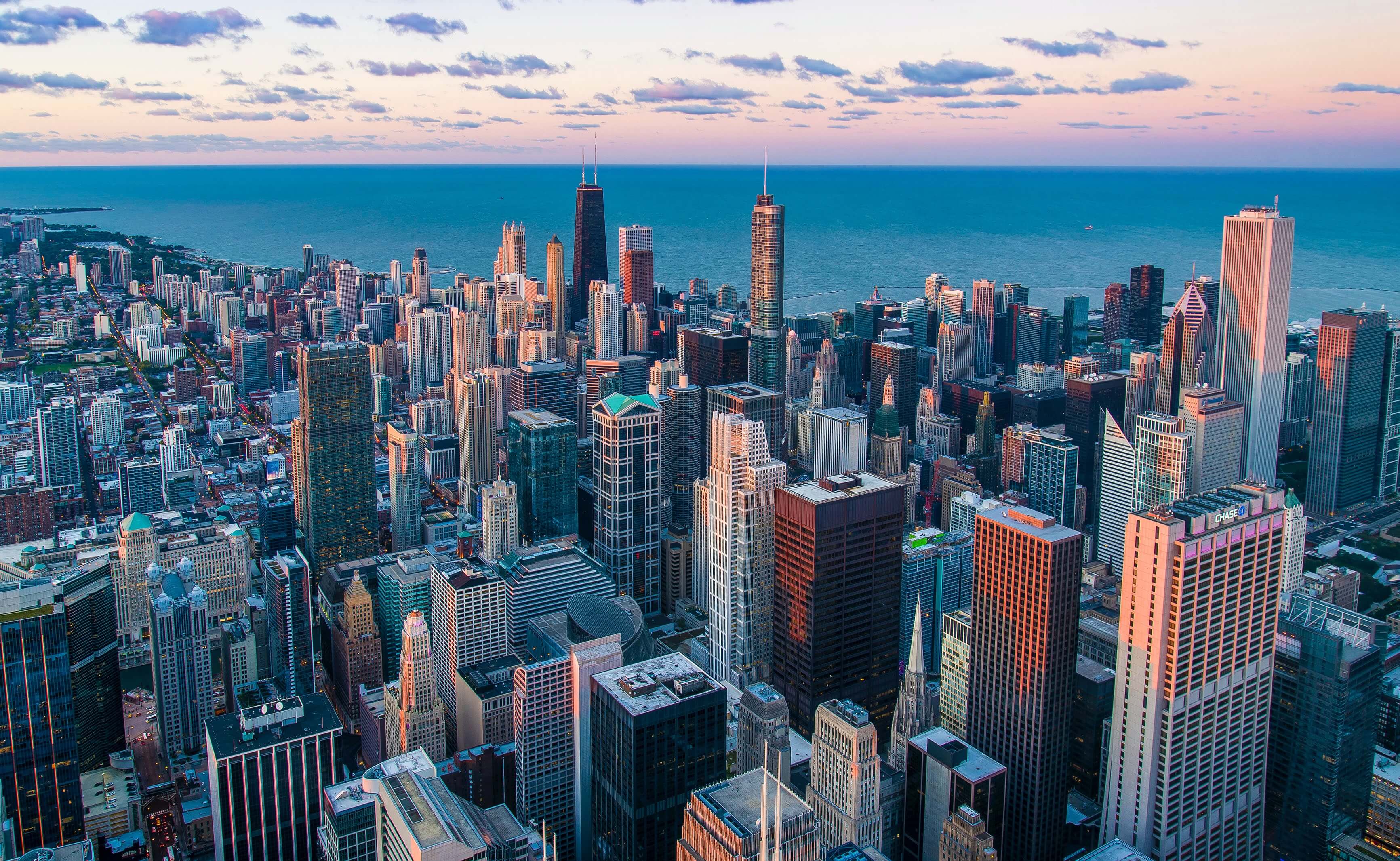
ASP NET Core 3 HTTP Patch
Understand JSON Patch
ASP.NET Core has support for working with the JSON Patch standard, which allows changes to be specified in a uniform way. The JSON Patch standards allows for a complex set of changes to be described. For more information about the details of you can visit https://toolds.ietf.org/html/rfc6902
Here is an example of a HTTP PATCH Request.
[
{ "op": "repalce",
"path": "Name",
"value": "Foo"
},
{
"op": "repalce",
"path": "City",
"value": "Bar"
}
]
A JSON Patch document is expressed as an array of operations. Each operation has an op property, which specifies the type of operation, and a path property, which specifies where the operation will be applied.
The JSON Patch document above sets new values for the Name and City properties considering our class below.
public class Foo
{
public string Name { ... }
public string City { ... }
}
Installing and configuring the JSON Patch Package
The Microsoft implementation of JSON Patch relies on the third-party Newtonsoft JSON.NET serializer that was used in ASP NET Core 2.x but that has been replaced with a bespoke JSON serializer in ASP NET Core 3.x.
dotnet add package Microsoft.AspNetCore.Mvc.NewtonsoftJson
Enabled the JSON.NET Serializer in the Startup.cs file.
services.AddNewtonsoftJson();
Defining your controller endpoint
[HttpPatch("{id}"]
public async Task<Foo> PatchFoo(int id, JsonPatchDocument<Foo> patchDoc)
{
var foo = await context.Foos.FindAsync(id);
patchDoc.ApplyTo(foo);
await context.SaveChangesAsync();
return foo;
}
The action method is decorated with the HttpPatch attribute, which denotes that it will handle HTTP requests. The model binding feature is used to process the JSON Patch document through a JsonPatchDocument<T> method parameter. The JsonPatchDocument<T> class defines an ApplyTo method, which applies each operation to an object.